home.gno
11.03 Kb · 252 lines
1package home
2
3import (
4 "std"
5 "strings"
6
7 "gno.land/p/demo/ufmt"
8 "gno.land/r/dragan/config"
9)
10
11type SocialLink struct {
12 URL string
13 Text string
14}
15
16type Project struct {
17 Name string
18 Description string
19 URL string
20 ImageURL string
21}
22
23var (
24 aboutMe string
25 socialLinks map[string]SocialLink
26 gnoProjects map[string]Project
27 web3Projects map[string]Project
28 otherProjects map[string]Project
29 textArt string
30 comingSoon string
31)
32
33func init() {
34 aboutMe = "I am a final-year student of Applied Software Engineering at the Faculty of Technical Sciences in Novi Sad, with a strong focus on blockchain and Web3 technologies.\n\n Since attending the Web3 Camp in Petnica, I have been actively exploring decentralized systems, smart contract development, and blockchain infrastructure.\n\n Additionally, I have experience in AI, particularly in NLP and neural networks. I have competed in and won multiple hackathons, including ETH Sofia, where I secured four sponsor bounties. Passionate about contributing to the blockchain ecosystem, I am eager to collaborate on innovative projects and continue expanding my expertise.\n\n"
35 comingSoon = "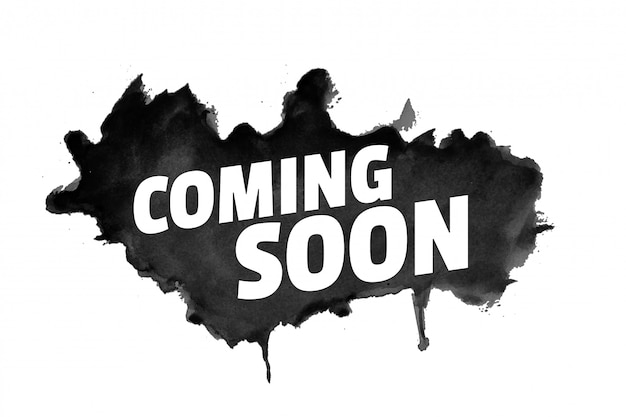\n"
36
37 socialLinks = map[string]SocialLink{
38 "GitHub": {URL: "https://github.com/Milosevic02", Text: "Explore my repositories and open-source contributions."},
39 "LinkedIn": {URL: "https://www.linkedin.com/in/dragan-milosevic3/", Text: "Connect with me professionally."},
40 "Email Me": {URL: "mailto:milosevicdragan002@gmail.com", Text: "Reach out for collaboration or inquiries."},
41 }
42
43 gnoProjects = make(map[string]Project)
44 web3Projects = make(map[string]Project)
45 otherProjects = make(map[string]Project)
46
47 gnoProjects["Liberty Bridge"] = Project{
48 Name: "Liberty Bridge",
49 Description: "Liberty Bridge was my first Web3 project, developed as part of the Web3 Bootcamp at Petnica. This project served as a centralized bridge between Ethereum and gno.land, enabling seamless asset transfers and fostering interoperability between the two ecosystems.\n\n The primary objective of Liberty Bridge was to address the challenges of connecting decentralized networks by implementing a user-friendly solution that simplified the process for users. The project incorporated mechanisms to securely transfer assets between the Ethereum and gno.land blockchains, ensuring efficiency and reliability while maintaining a centralized framework for governance and operations.\n\n Through this project, I gained hands-on knowledge of blockchain interoperability, Web3 protocols, and the intricacies of building solutions that bridge different blockchain ecosystems.\n\n",
50 URL: "https://gno.land",
51 ImageURL: "https://github.com/Milosevic02/LibertyBridge/raw/main/lb_banner.png",
52 }
53
54 gnoProjects["Liars Lie"] = Project{
55 Name: "Liars Lie",
56 Description: "",
57 URL: "https://gno.land",
58 ImageURL: "https://img.freepik.com/free-vector/abstract-grunge-style-coming-soon-with-black-splatter_1017-26690.jpg\n",
59 }
60
61 web3Projects["Incognito"] = Project{
62 Name: "Incognito",
63 Description: "Incognito is a Web3 platform built for Ethereum-based chains, designed to connect advertisers with users in a privacy-first and mutually beneficial way. Its modular architecture makes it easily expandable to other blockchains. Developed during the ETH Sofia Hackathon, it was recognized as a winning project for its innovation and impact.\n\nThe platform allows advertisers to send personalized ads while sharing a portion of the marketing budget with users. It uses machine learning to match users based on wallet activity, ensuring precise targeting. User emails are stored securely on-chain and never shared, prioritizing privacy and transparency.\n\nWith all campaign data stored on-chain, Incognito ensures decentralization and accountability. By rewarding users and empowering advertisers, it sets a new standard for fair and transparent blockchain-based advertising.\n\nDemo video of the project can be viewed at this link: [Incognito Demo Video](https://dorahacks.io/buidl/17725)",
64 URL: "https://github.com/Milosevic02/Incognito-ETHSofia",
65 ImageURL: "https://i.ibb.co/cS6RPW9L/Screenshot-2025-02-05-084304.png",
66 }
67
68 web3Projects["Solidity Exploits Research"] = Project{
69 Name: "Solidity Exploits Research",
70 Description: "From August 1 to 10 2024, I had the incredible opportunity to attend the Web3 Camp in Petnica Science Center, where I worked on a research project focused on Solidity exploits. This project explored vulnerabilities in smart contracts, including reentrancy attacks, front-running, and integer overflows. The experience provided deep insights into blockchain security and best practices for writing secure Solidity code.\n\nYou can check out the presentation here: [Solidity Exploits Research](https://docs.google.com/presentation/d/1LziLmONP9oLpyRU5SrKUBCVqJ3yY1gOC/edit#slide=id.p1).",
71 URL: "https://docs.google.com/presentation/d/1LziLmONP9oLpyRU5SrKUBCVqJ3yY1gOC/edit#slide=id.p1",
72 ImageURL: "https://i.ibb.co/1t00VYMJ/Dragan-Milosevic-Solidity-Exploits-pptx.png", //Fix this
73 }
74
75 otherProjects["OtherProjects"] = Project{
76 Name: "Highlight Projects",
77 Description: "→ **BankBot - COMTRADE EDIT Hackathon - First Place** Smart banking chatbot built with Dialogflow, BERT, LangChain, and ChatGPT API. [Presentation](https://drive.google.com/file/d/11IDrka4hjhUCLfdF0EHRVgBx_YH-XSxi/view).\n\n" +
78 "→ **Full-Stack Web Application Development**: Developed **Šped Helper** for a client, a full-stack app designed for a freight and shipping company, with business-critical functionalities.\n\n" +
79 "→ **HardSkills Academy Hackathon - First Place**: Created a model for land cover classification using satellite images.\n\n" +
80 "→ **BOSCH Future Mobility Challenge (Nov 2023 - Mar 2024)**: Led autonomous vehicle development, focusing on navigation and obstacle detection algorithms.\n\n" +
81 "→ **Ethical Hacking Projects**: Developed ethical hacking tools, including a screenshot malware and ransomware simulation.\n\n" +
82 "→ **Artificial Intelligence & Neural Networks**: Worked on various AI and NN projects as part of learning in the field.\n\n" +
83 "🔍For more details on these projects, feel free to check my [LinkedIn](https://www.linkedin.com/in/dragan-milosevic3/).",
84 URL: "https://www.linkedin.com/in/dragan-milosevic3/",
85 ImageURL: "",
86 }
87}
88
89func Render(path string) string {
90 var sb strings.Builder
91 sb.WriteString("# Hi, I'm Dragan \n\n")
92 sb.WriteString("---\n")
93 sb.WriteString("## 👋 About me\n")
94 sb.WriteString(aboutMe)
95 sb.WriteString("---\n")
96 sb.WriteString(renderProjects(gnoProjects, "⚙️ Gno Projects"))
97 sb.WriteString("---\n")
98 sb.WriteString(renderProjects(web3Projects, "🌐 Web3 Projects"))
99 sb.WriteString("---\n")
100 sb.WriteString(renderProjects(otherProjects, "📂 Other Projects"))
101 sb.WriteString("---\n")
102 sb.WriteString("## 🎮 Games\n")
103 sb.WriteString("### " + "⏳" + comingSoon + "\n")
104 sb.WriteString("---\n")
105 sb.WriteString(renderRewardsAndAchievements())
106 sb.WriteString(renderSocialLinks())
107
108 return sb.String()
109}
110
111func renderRewardsAndAchievements() string {
112 var sb strings.Builder
113 sb.WriteString("---\n")
114 sb.WriteString("## 🏅 Rewards & Achievements\n\n")
115 sb.WriteString("- 🏆 **ETH Sofia** – Won 4 sponsors’ bounties:\n")
116 sb.WriteString(" - 🥇 1st place – Chainbase, iExec, SEEBLOCKS.eu\n")
117 sb.WriteString(" - 🥉 3rd place – AI Open Track\n")
118 sb.WriteString("- 🏆 **First place** in the COMTRADE EDIT competition for creating a bank chatbot with NLU capabilities.\n")
119 sb.WriteString("- 🏆 **First place** in a hackathon on convolutional neural networks.\n")
120 sb.WriteString("- 🚀 **Attended** Web3 Camp in Petnica.\n")
121 sb.WriteString("- 🌟 **Participated** in the BOSCH FUTURE MOBILITY CHALLENGE.\n")
122 sb.WriteString("---\n")
123
124 return sb.String()
125}
126
127func renderSocialLinks() string {
128 var sb strings.Builder
129 sb.WriteString("## 🔗 Links\n\n")
130 sb.WriteString("You can find me here:\n\n")
131 sb.WriteString(ufmt.Sprintf("- 🐙 [GitHub](%s) - %s\n", socialLinks["GitHub"].URL, socialLinks["GitHub"].Text))
132 sb.WriteString(ufmt.Sprintf("- 👜 [LinkedIn](%s) - %s\n", socialLinks["LinkedIn"].URL, socialLinks["LinkedIn"].Text))
133 sb.WriteString(ufmt.Sprintf("- 📧 [Email Me](%s) - %s\n", socialLinks["Email Me"].URL, socialLinks["Email Me"].Text))
134 sb.WriteString("\n")
135 return sb.String()
136}
137
138func renderProjects(projectsMap map[string]Project, title string) string {
139 var sb strings.Builder
140 sb.WriteString(ufmt.Sprintf("## %s\n\n", title))
141 for _, project := range projectsMap {
142 sb.WriteString(ufmt.Sprintf("### [%s](%s)\n\n", project.Name, project.URL))
143 if project.ImageURL != "" {
144 sb.WriteString(ufmt.Sprintf("\n\n", project.Name, project.ImageURL))
145 }
146 sb.WriteString(project.Description + "\n\n")
147 }
148 return sb.String()
149}
150
151func UpdateLink(name, newURL string) {
152 if !isAuthorized(std.PreviousRealm().Address()) {
153 panic(config.ErrUnauthorized)
154 }
155
156 if _, exists := socialLinks[name]; !exists {
157 panic("Link with the given name does not exist")
158 }
159
160 socialLinks[name] = SocialLink{
161 URL: newURL,
162 Text: socialLinks[name].Text,
163 }
164}
165
166func UpdateAboutMe(text string) {
167 if !isAuthorized(std.PreviousRealm().Address()) {
168 panic(config.ErrUnauthorized)
169 }
170
171 aboutMe = text
172}
173
174func AddGnoProject(name, description, url, imageURL string) {
175 if !isAuthorized(std.PreviousRealm().Address()) {
176 panic(config.ErrUnauthorized)
177 }
178 project := Project{
179 Name: name,
180 Description: description,
181 URL: url,
182 ImageURL: imageURL,
183 }
184 gnoProjects[name] = project
185}
186
187func DeleteGnoProject(projectName string) {
188 if !isAuthorized(std.PreviousRealm().Address()) {
189 panic(config.ErrUnauthorized)
190 }
191
192 if _, exists := gnoProjects[projectName]; !exists {
193 panic("Project not found")
194 }
195
196 delete(gnoProjects, projectName)
197}
198
199func AddWeb3Project(name, description, url, imageURL string) {
200 if !isAuthorized(std.PreviousRealm().Address()) {
201 panic(config.ErrUnauthorized)
202 }
203 project := Project{
204 Name: name,
205 Description: description,
206 URL: url,
207 ImageURL: imageURL,
208 }
209 web3Projects[name] = project
210}
211
212func RemoveWeb3Project(projectName string) {
213 if !isAuthorized(std.PreviousRealm().Address()) {
214 panic(config.ErrUnauthorized)
215 }
216
217 if _, exists := otherProjects[projectName]; !exists {
218 panic("Project not found")
219 }
220
221 delete(web3Projects, projectName)
222}
223
224func AddOtherProject(name, description, url, imageURL string) {
225 if !isAuthorized(std.PreviousRealm().Address()) {
226 panic(config.ErrUnauthorized)
227 }
228 project := Project{
229 Name: name,
230 Description: description,
231 URL: url,
232 ImageURL: imageURL,
233 }
234 otherProjects[name] = project
235}
236
237func RemoveOtherProject(projectName string) {
238 if !isAuthorized(std.PreviousRealm().Address()) {
239 panic(config.ErrUnauthorized)
240 }
241
242 if _, exists := otherProjects[projectName]; !exists {
243 panic("Project not found")
244 }
245
246 delete(otherProjects, projectName)
247}
248
249func isAuthorized(addr std.Address) bool {
250
251 return addr == config.Address() || addr == config.Backup()
252}